Building an App Series Part 4: Variable Assignments and Data Structures

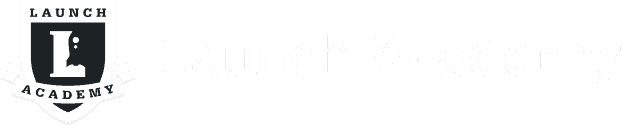
If you have been reading the CodeBuddy series attentively, you are probably thinking, “Dude! Let’s start coding! Enough with all the meta talk!” Well grasshopper, there are a few things you absolutely need to know before you can start programming in Ruby. How do you expect to be an excellent Ruby programmer without at least some exposure to Ruby’s core functionality? Simply put, you can’t.
In the last article, we discussed what it is like to develop software in Ruby from a more meta level. We also introduced you to simple Ruby objects like strings
, integers
, and floats
. In this article, we will discuss how Ruby developers organize these objects. You will learn the best practices pertaining to assigning and naming your variables, as well as best use cases for Ruby’s data structures.
Variable Assignment and Naming Conventions
In Ruby, we assign objects like strings
, integers
, and floats
to variables to use throughout the various methods in our applications. In the last article, we talked about manipulating objects with instance methods. We had to call the object itself every time we wanted to do something to it.
But, what if that object is really long or complicated? Why should we have to type out the entire thing? The best way to explain variable assignment is to break down some examples. Take note of how we name variables to represent the content of each object.
fox_string = “The quick brown fox jumped over the lazy dog.”
fox_string
=> “The quick brown fox jumped over the lazy dog.”
fox_string.reverse
=> “.god yzal eht revo depmuj xof nworb kciuq ehT”
sum = 5 + 6
sum
=> 11
sum - 2
=> 9
As developers, assigning variables to lengthy strings and data structures allows us to work faster without having to repeat ourselves. If I want to manipulate an object, calling the variable it is assigned to is far more conducive to developing highly effective software as efficiently as possible.
Imagine another developer working on your project and they needed to make an adjustment to certain components. As developers we have to be diligent about naming variables so as not to confuse objects. Lazy developers are known to write cryptic, unintuitive variables when writing software. Deciphering the meaning of a variable is a waste of time.
#DON’T DO THIS!
broccoli = 5
cats = “Shiba Inu”
Why on Earth would we assign an integer to the variable broccoli
? Why would we assign a dog breed to the variable cats
? Closely aligning a variable’s name with its contents is a key component to being a successful developer. No one wants to work on a project with cryptic, unintelligible variables.
To learn more about intelligent variable assignments in Ruby, check out this awesome Ruby style guide on GitHub.
Data Structures in Ruby
Most programming languages utilize various types of data structures in order to organize objects. In Ruby, developers usearrays and hashes to organize data structures. In this section, we will explain how developers use these data structures and their respective best practices.
Arrays
Arrays are objects that contain sets of data. As developers, we use arrays to maintain collections of objects. Arrays have their own set of instance methods that can be called upon them.
There are a few ways to initialize a new array.
new_array = Array.new
=> []
empty_array = []
=> []
random_objects_array = [“buckets”, 3.14, 75]
=> [“buckets”, 3.14, 75]
In the first two examples, the empty brackets represent the persistence of an empty array. In the third example (random_objects_array
), we created an array by inputting 3 separate objects within the brackets, separated by commas. The commas separate the objects from one another. Notice how there are three different types of objects.
What if we wanted to call on one particular object in array? Let’s look at random_objects_array
.
random_objects_array = [“buckets”, 3.14, 75]
random_objects_array[0]
=> “buckets
random_objects_array[1]
=> 3.14
random_objects_array[2]
=> 75
In order to call individual elements of an array, we use the array’s index. The index of the first element is always 0, and goes up as we progress through an array’s elements.
So now we can identify elements in an array starting from the beginning. What if we wanted to start from the end?
random_objects_array = [“buckets”, 3.14, 75]
random_objects_array[-1]
=> 75
random_objects_array[-2]
=> 3.14
In Ruby, we use negative integers to identify indices starting from the end of an array. The last element in an array will always have an index of -1.
There are dozens of methods available for sorting and manipulating arrays. Check out the Ruby Docs section on Arrays for a list of methods and how they’re applied in Ruby code.
Hashes
In Ruby, developers use hashes to organize data pairs. Each pair has a unique key and associated value. Syntactically, a hash uses curly braces to define its endpoints. Let’s take a look at a few examples to better understand their structure.
{ “Mikey” => 19 }
{ “Tommy” => { “parents” => [“Andrew”, “Megan”] } }
ages = { “Vikram” => 34, “Dan” => 30, “Richard” => 31, “Ellen” => 21 }
With arrays, we use an element’s index to isolate it. However, with hashes we use the element’s key to isolate its value.
ages = { “Vikram” => 34, “Dan” => 30, “Richard” => 31, “Ellen” => 21 }
ages[“Vikram”]
=> 34
ages[“Ellen”]
=> 21
ages[“Vikram”] + ages[“Ellen”]
=> 55
When we start building CodeBuddy, we will be utilizing both arrays and hashes to organize Reference
objects. To learn more about hashes and methods we can call on them, check out the Ruby Docs section on Hashes.
Once you have become comfortable with the basics of Ruby data structures, check out the Rubies gem to practice working with more complex arrays and hashes. Follow the instructions in the README for installation instructions.
Working with variables and data structures is a major component of developing in any programming language, especially Ruby. The only way to get better with them is to practice working with them every day until it gets easier.
This was definitely an abridged introduction to these concepts. With that in mind, the following are great resources for getting comfortable with assigning variables and complex data structures in Ruby.
Next time, we will discuss flow control in Ruby, in addition to boolean values.
Challenges
Array Challenges
complex_array = [[1, 3, 5, 6], [7, 8, 9], 2]
- How would you output the integer `6`?
- Replace the integer `2` to an array with the numbers `[2, 4, 12, 24, 36]`.
Hash Challenges
characters = {
"Omar Little" => {
"friends" => ["Butchie", "Jimmy McNulty"],
"enemies" => ["Avon Barksdale", "Stringer Bell", "Marlo Stanfield", "Chris Partlow", "Snoop Pearson"],
},
"Lester Freamon" => {
"friends" => ["Roland Pryzbylewski", "Jimmy McNulty", "Bunk Moreland", "Cedric Daniels"],
"hobbies" => ["crafting model furniture", "solving crimes", "putting drug dealers in jail"]
}
}
Given the above data structure, how would you output:
- Omar Little’s enemies
- Lester Freamon’s hobbies
- Snoop Pearson
- Jimmy McNulty