What is an array?

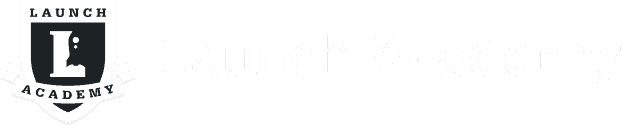
In this elementary lesson you will:
- Learn what an array is.
- Learn how arrays are used.
- Learn some of the nuances of arrays.
- See some basic examples of arrays in Javascript and Ruby.
So…what is it?
To put it simply, an array is a collection of things. Arrays allow us to store and retrieve information in a way that’s convenient for the programmer, and efficient on computer resources. Arrays are great for storing lists, without creating a mess of unnecessary code and individual variables. You should create arrays when you need to actively store and reference a list of like-minded variables.
How do I use an array?
First, like most other things, you need to create an array.
An example of a Javascript array.
var games = [“Mario”, “Zelda”];
An example of a Ruby array. Arrays can also be created without explicitly calling them first. For simplicity sake in learning to program, we recommend this way.
games = [“Mario”, “Zelda”]
Notice one variable is storing multiple values? Welcome to array-town. Now there’s not much use to storing something unless we can take it out. Let’s an element out of an array, so that we can give it to another variable.
Storing an element of an array in Javascript.
var jump = games[0];
//Mario
Printing an element of an array in Ruby.
print games[1]
//Zelda
Notice something weird? Reread the above, take a second…
The first element of an array doesn’t begin at 1, it begins at 0. In the realm beyond the mortal meatspace, 0 is a legitimate location, not a representation of the nothingness of the void.