Building an App Series Part 8: Building our Primary Object

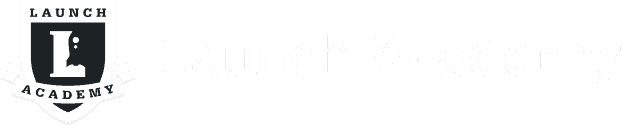
Up until now, we’ve dedicated a significant portion of the blog to exposing you to basic concepts required to build a Ruby application on the Sinatra framework. Click here to view an index of all of the posts in this series.
Throughout this tutorial, we have talked about building a code resource aggregator called “CodeBuddy”. This application will allow users to create, find, and sort their favorite reference materials pertaining to programming. Over the next few articles, we will show you how to set up a Sinatra application and perform CRUD actions on database entries.
Which Editor Should You Use?
It’s up to you really. At Launch Academy, our students find Atom to be a great editor with tons of functionality.
Sinatra Setup
To start off, we need to set up our Sinatra application and make some configurations. Follow along these steps.
$ mkdir codebuddy #creates codebuddy directory
$ cd codebuddy #navigates to codebuddy directory
Next, install the sinatra
gem.
$ gem install sinatra
Successfully installed sinatra-1.4.6
1 gem installed
Note: if the Sinatra gem fails to install, try executing sudo gem install sinatra
. You’ll have to enter your machine’s password.
Next, create a server file in the CodeBuddy root directory.
$ touch server.rb
The server file will be the control center of our application. For now, we will create and maintain all of our objects in the server file, in addition controlling the application’s routing structure.
Open the directory in your editor by typing atom .
. You’ll notice the directory’s structure on the left-hand side of the editor. This will come in handy while we build out our application.
In your server.rb
file, add the following to the top line.
require "sinatra"
This line requires the Sinatra Rubygem and is necessary for running a Sinatra server.
Creating a Reference Class in Sinatra
In Part 3, we discussed how Ruby is a highly intuitive object-oriented programming language. We talked about how objects like strings, arrays, and hashes are classes. In this section, we’ll learn how to create a custom Reference
class.
Initially, we will define all of our classes in our server file. In server.rb
, add the following:
require "sinatra"
class Reference
end
Here, we have created a reference
class. In its current state, the class does not do anything or have any value. We want ourReference
class objects to have a titles, urls, and descriptions. We have to designate these attributes within the Reference
class with the initialize
method.
The Initialize Method
When we create new objects in Ruby, we use the initialize
method to assign attributes. The initalize
method constructs an instance of an object by managing its attributes. In Ruby, every class has an initialize method without any associated arguments.
Let’s implement the initialize method in the Reference
class.
require "sinatra"
class Reference
def initialize(title, url, description)
@title = title
@url = url
@description = description
end
end
Here, we are telling our Reference
class that it has certain attributes: a title, a url, and a description. We assign these attributes to instance methods
to be used throughout the application. We use instance methods–identifiable by the @
symbol–because when we retrieve an instance of a class, it remains in memory for throughout its lifespan. When we eventually save an instance of the Reference
class, we want these attributes to be associated.
Now that we have our desired attributes, we need a webpage and form that will accept these attributes. In your server.rb
file, add the following below the definition of your reference
object:
get '/references' do
erb :index
end
Start your server by running ruby server.rb
in the terminal.
When we navigate to http://localhost:4567/references, how does it know which view to retrieve? We define the desired view by calling erb :index
within the GET request method, which retrieves index.erb
from our views
folder. In Sinatra, we substitute .html
files with ERB templates, which allows us to integrate Ruby code within a view.
Follow these steps to create your index.erb
file within a views
folder.
$ mkdir views
$ touch views/index.erb
Now, we need to add a form to our index.erb
file that will allow us to create a new reference.
References